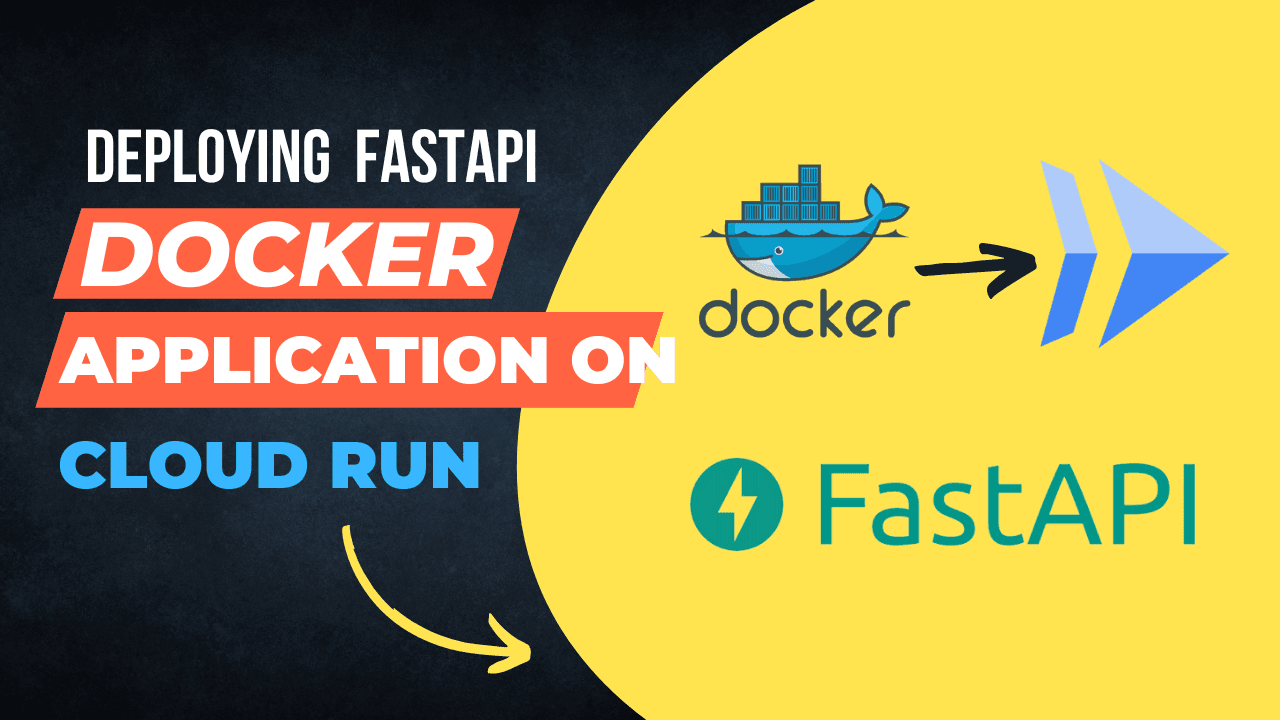
Dockerizing your application allows you to create a container image that can be deployed on Cloud Run. To deploy your FastAPI application on Cloud Run, you must create a container image using Docker. A container image is a lightweight package that includes your application and its dependencies.
Project Setup:-
To set up a FastApi application that is deployable on a cloud run, Set up a virtual environment (optional but recommended):.
python3 -m venv venv
source venv/bin/activate
Now install FastAPI and Uvicorn(Uvicorn is an ASGI web server implementation for Python).
$ pip install fastapi
$ pip install 'uvicorn[standard]' (minimal installation)
Now create a minimal FastAPI application by creating a main.py inside an app directory as below.
from typing import Union
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def read_root():
return {"Hello": "World"}
@app.get("/recipe/{recipe_id}")
async def read_recipe(recipe_id: int, q: Union[str, None] = None):
return {"recipe_id": recipe_id, "q": q}
Now you have a basic FastAPI project set up. You can define more routes and build out your application logic within the main.py file. FastAPI also provides powerful features such as automatic request validation, data serialization, and support for asynchronous code, making it a great choice for building web APIs.
Note: In this article, we will be focused on Deploying FastAPI Dockerize Application on Cloud Run instead of building and understanding the concept of FastAPI.
Now create a .env file in the root directory of your project and add the below environment variables in the file. These variables will be used by google cloud run.
GCP_CLOUD_PROJECT="cloud-kota"
GOOGLE_APPLICATION_CREDENTIALS='/gcp/creds.json' # optional if you need access to other services from cloud run
PORT='80'
K_SERVICE='prod'
Build Docker Image:-
Now, in your FastAPI application's root directory, create a docker file as Dockerfile.
FROM python:3.9
# create directory for your application in the docker container.
WORKDIR /honey-food
# copy your requirements.txt file in the docker container.
COPY ./requirements.txt /honey-food/requirements.txt
# install dependencies in the docker container using requirements.txt
RUN pip install --no-cache-dir --upgrade -r /honey-food/requirements.txt
# copy your current ./app(application source code) into docker container
COPY ./app /honey-food/app
# copy your environement variables file into docker container
COPY ./.env /honey-food/.env
CMD ["uvicorn", "app.main:app", "--host", "0.0.0.0", "--port", "80"]
Now use the Dockerfile to build the Docker image of your FastAPI application. Open a terminal or command prompt in the directory containing the Dockerfile and run the following command:
docker build -t honey-food ./app
To run your fast API docker application locally you can run the below command.
docker run -p 80:80 \
--env-file .env \
# copying creds json from local machine to docker container
-v "$HOME/.config/gcloud/application_default_credentials.json":/gcp/creds.json:ro \
--name honey-food \
kodeweich/honey-food
Push the Docker image to a container registry:
Before deploying to Cloud Run, you need to push the Docker image to a container registry such as Google Container Registry (GCR). Create a repository in the GCR and Run the below command to authenticate with your GCR repository from your terminal.
gcloud auth configure-docker asia-south1-docker.pkg.dev
Now tag your image and push the docker image to the container registry using the below command.
# tag docker image
docker tag <local_image_name> asia-south1-docker.pkg.dev/<project_id>/<remote_image_name>
# push docker image
docker push asia-south1-docker.pkg.dev/<project_id>/<remote_image_name>:latest
Deploy Application on Cloud Run:
To run your FastAPI application on the cloud run the below command.
gcloud run deploy honey-food --image asia-south1-docker.pkg.dev/<project_id>/<remote_image_name>:latest
Once the deployment is successful, you will see the URL of your Cloud Run service. You can access your FastAPI application by opening that URL in a web browser or using cURL or any HTTP client.
Conclusion:
In this article, we see that to deploy a FastAPI application on Google Cloud Run, build a Docker image with the application code and dependencies, push it to a container registry like GCR, and create a Cloud Run service using the image. The service provides scalability and can be accessed using the provided URL, integrating seamlessly with Google Cloud infrastructure.